How to add css to wp_head
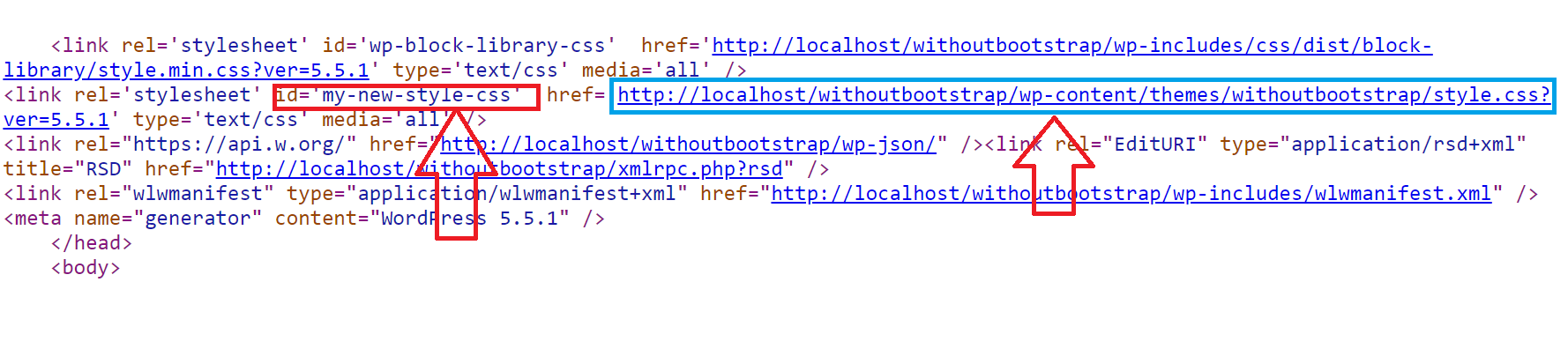
When you edit the child theme or standard theme, you will see stylesheet links tags that came with a theme.
Always a standard theme retrieves the stylesheet by wp_head function.
And so, the same function is able to include your own custom stylesheet.
In fact, you can add CSS to wp_head. Hence wp_head in the child theme will add your own stylesheet in the pages and blog categories.
To add CSS to wp_head, define a new function in functions.php, in the new function call wp_enqueue_style() and pass two arguments in the wp_enqueue_style. The two arguments are the name of the CSS file and the path of the stylesheet file.
Therefore, you will need a series of WordPress functions to add the stylesheet. These WordPress functions are
- wp_enqueue_style
- get_stylesheet_uri
- add_action
Add the custom CSS using wp_enqueue_style.
wp_enqueue_style function enqueues the custom css, I found this is very useful to avoid the repeating of the stylesheet.
To add custom css using wp_enqueue_style requires to pass parameters, which are two strings for a style name and the path of the CSS file.
In order to load a custom stylesheet.
open functions.php file.
Create a new function, I name the function as add_custom_stylesheet.
1.function add_custom_stylesheet()
2.{
Call wp_enqueue_style, and pass two arguments in this function.
The first argument is the stylesheet name like ‘the-style-name’ and the URI of the CSS file.
You need to use get_template_directory_uri() to retrieve the current theme directories.
Then append the stylesheet name to the function.
3.wp_enqueue_style("the-style-name",get_template_directory_uri(). '/'. 'mycustom-stylesheet.css');
4.}
Call add_action hook to include CSS to wp_head
5.add_action('wp_enqueue_scripts', ' add_custom_stylesheet');
These few lines of codes will output the stylesheet link tag using the wp_head function.
But these lines add only one CSS file although it is possible to customize add_custom_stylesheet
to include many stylesheets at once.
If you want to know how to do it, check out the next section.
Adding Multiple CSS files to wp_head
Imagine you have created at least two CSS files that serve different purposes.
You may want to include them to wp_head, aren’t you?
To add multiple CSS files in the WordPress theme, create a new function in the functions.php file.
In this example, my function name is multiple-css-file
1.function multiple-css-file()
2.{
Call wp_enqueue_style and pass the string parameters of the stylesheet name and CSS path.
Write wp_enqueue_style multiple times, depending on the number of CSS you want to add, like this.
4.wp_enqueue_style("the-style-name-1",get_template_directory_uri(). '/'. 'mycustom-stylesheet-1.css');
5.wp_enqueue_style("the-style-name-2",get_template_directory_uri(). '/'. 'mycustom-stylesheet-2.css');
5.wp_enqueue_style("the-style-name-3",get_template_directory_uri(). '/'. 'mycustom-stylesheet-3.css');
6.wp_enqueue_style("the-style-name-4",get_template_directory_uri(). '/'. 'mycustom-stylesheet-4.css');
7.}
8.add_action('wp_enqueue_scripts', ' multiple-css-file');
When you reload the page, you will see all the CSS files you have added.
Add CSS to a specific page to wp_head.
WordPress provides the capability to load different CSS for different pages.
You need to use conditional statements in functions.php. The conditional statement tests the is_page function.
The scenario happens when you want different designs for different pages.
To add CSS to a specific page or category in WordPress, write the following codes in functions.php
1.function css-for-specific-page(){
2.if(is_page('About')){
3.wp_enqueue_style("about-page",get_template_directory_uri(). '/'. 'about-page.css');
4.}//close if statement
5.}//close a function
6.add_action('wp_enqueue_scripts', 'css-for-specific-page');
In fact, there are few steps to follow to add a CSS file to a specific page.
These are steps I followed to write the code for adding different css files to different pages.
Create a function in the functions.php
I named this function css-for-specific-page.
Including the stylesheet using WordPress functions, it is the most proper way.
That is what I have learned from the answers of StackOverflow for the questions relating to this issue.
Although, a CSS file can be added directly by writing a stylesheet link tag in the page.php file.
But the recommendation for best practice is to enqueue the stylesheet by wp_enqueue_style.
Now, here it is the actual function.
1.function css-for-specific-page(){
Then,
Specify a page to add a CSS file.
This form a second line where you will write a conditional statement that checks a page or a category to add the stylesheet.
A conditional statement will check is_page()
function.
is_page()
function, as it was described in the WordPress developer website, determine the query for a specific single page.
The function receives an argument which you will use to define a single page to include the stylesheet.
The parameter can be a page name, page id, an array of page id, or an array of page names.
This is a required conditional statement to name a specific page to add a stylesheet.
2.if(is_page('About')){
After checking the page, then . . ,
Include a CSS file using wp_enqueue_style
After specifying the page that you want to add the stylesheet, the next line will add the actual CSS file.
This file will be enqueued by wp_enqueue_style.
The wp_enqueue_style will receive two parameters.
For this case, pass a string for the stylesheet name as the first parameter and stylesheet path as a second parameter,
This is how to do it.
3.wp_enqueue_style("about-page",get_template_directory_uri(). '/'. 'about-page.css');
4.}//close if statement
5.}//close a function
After that,
Execute the function by calling the add_action hook
To add the function to the wp_head, use the add_action function.
To properly execute add_action for the css-for-specific-page,
pass two strings.
- The action hook to add the script ie
wp_enqueue_scipts
- The name of the function to be executed ie
css-for-specific-page
The actual code is
6.add_action('wp_enqueue_scripts', 'css-for-specific-page');
Alternative of wp_head to add the CSS file
Instead of adding the CSS styles to wp_head, you can add them to the header.php file directly.
With header.php, you can include the CSS for
- specific page
- all blog pages
For example, if you want to specify a page that you want to add its own CSS, you will write the conditional statements which check the is_page function.
Then,
Write a stylesheet link tag you want as the following codes explain.
<head>
1.<?php if(is_page('contact')){
2.echo '<link rel="stylesheet" href="style1.css">';
3.}else if(is_page('about')){
4.echo '<link rel="stylesheet" href="style2.css">';
5.}?>
</head>
But, I don’t like to include the CSS files in the WordPress theme files when editing a theme developed by someone else.
I always use the wp_enqueue_style function to avoid CSS files duplication.
Wrap-up
To add CSS stylesheet to wp_head can be achieved by creating a function which enqueues stylesheet in the functions.php.
The function adds the stylesheet to wp_head using the wp_enqueues_style, with wp_enqueue_style you can add multiple stylesheets and a stylesheet to the specific page.