WordPress functions to add internal and external stylesheet
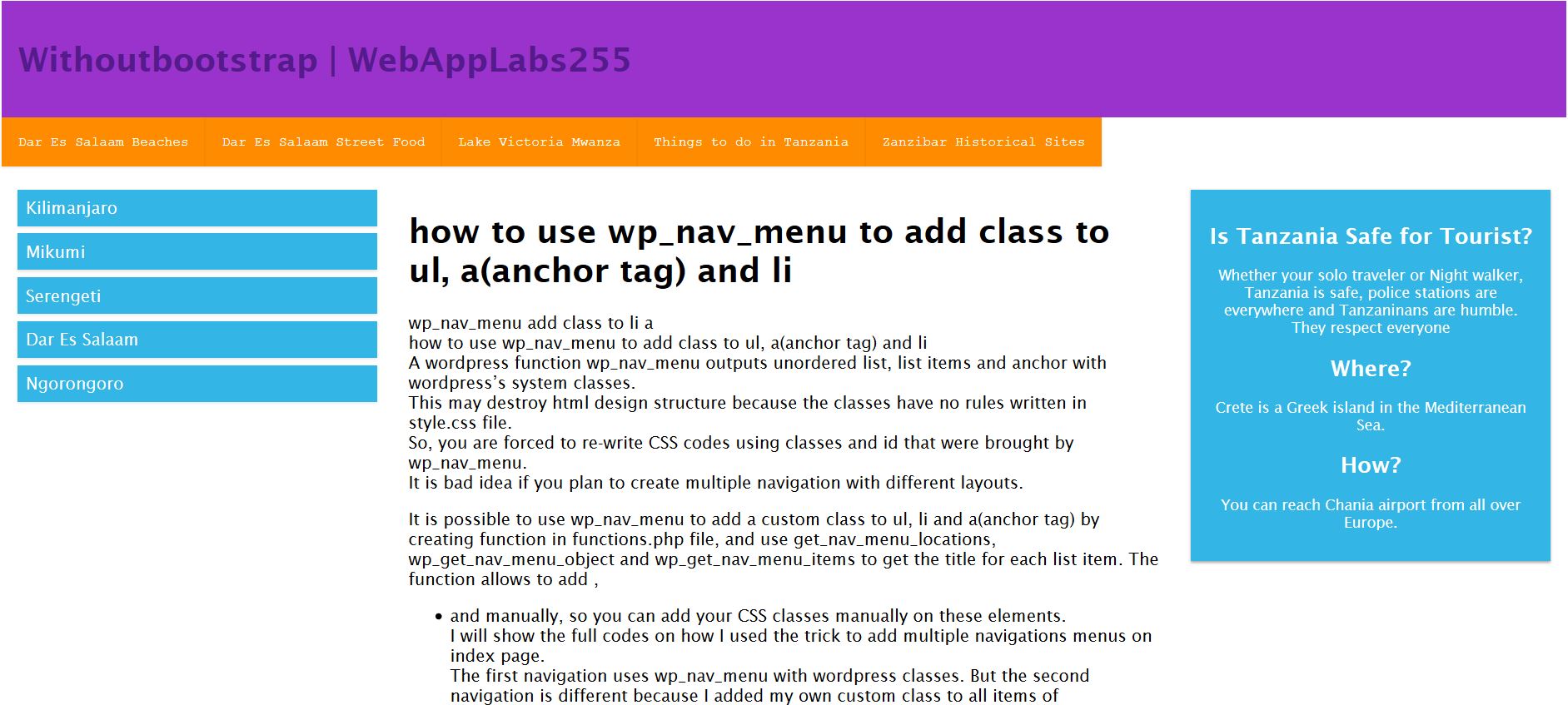
The first step during WordPress theme development is template pages designing.
A page layout presentation is styled by CSS stylesheet.
But an index.php and header.php won’t load style.css file when pages are moved to the theme directory, this destroys the HTML page structure.
The page site title function won’t bring pretty looking blog title, and the wp_nav_menu function returns an unstyled menu.
It was confusing me before finding the solution.
The best solution to fix this is to call some WordPress functions to add a stylesheet to the respective theme files.
To include a stylesheet in the WordPress theme, use either wp_enqueue_style()
or bloginfo('stylesheet_url')
to add the CSS link tag in Html head. The wp_enqueue_style is called in the functions.php file while bloginfo('stylesheet_url')
is used in the CSS link tag.
Although, you can include stylesheets directly by writing a link tag. But, it is not the best practice at all.
You will find out why after understanding the uniqueness of wp_enqueue_style().
Adding internal stylesheet by wp_enqueue_style()
wp_enqueue_style adds a CSS file by enqueueing the provided CSS stylesheet. So, it is able to prevent CSS over-writing.
For example, my theme CSS stylesheet file is style.css
To enqueue style.css, first, create a function inside the functions.php file.
1.function adding-stylesheet(){
Then, call wp_enqueue_style by passing two parameters only.
The first parameter is a string for any stylesheet name you prefer. I will use the name ‘style’ for the first argument.
The second parameter is the path of the CSS file location. use get_stylesheet_url to retrieve the stylesheet URI located in the theme directory.
2.
wp_enqueue_style('
my-new-style',get_stylesheet_uri());
Then close a function by curly brackets (}).
To execute the adding-stylesheet function, use add_action function.
add_action takes two string parameters, which are the action tag specifying WordPress action hooks and the name of the function to be executed.
The WordPress action hook to be passed in add_action() for the wp_enqueue_style function is wp_enqueue_scripts
3.}
4.add_action('wp_enqueue_scripts', 'adding-stylesheet');
To load the stylesheet, open header.php or index.php and call wp_head()
function that is <?php wp_head();?>
Write the code line <?php wp_head();?>
in the HTML <head>
tag
So, if you reload the page you will see the change.
Here it is my page after using wp_enqueue_style
WordPress function to add a stylesheet.
You can also see a CSS name and stylesheet URI passed in wp_enqueue_style
function in the Html source.
The function receives five arguments and you can find other usages of wp_enqueue_style
in the WordPress developer website.
Adding an external stylesheet script.
To enqueue an external CSS script, use wp_enqueue_style
.
Then, pass a string name for the first argument and a URL, a link pointing to an external stylesheet, for example
wp_enqueue_style('external-url-name', 'https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css')
And use the add_action function to add the external stylesheet in Html pages.
The final step is to call wp_header in the index.php or header.php
This code below is a summary of how to include external stylesheet.
1.function include-external-stylesheet(){
2.wp_enqueue_style('my-new-style-2', 'https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css');
3.}
4.add_action(
'wp_enqueue_scripts','include-external-stylesheet')
Therefore, in order to render external CSS files, you must have the external URL of the stylesheet.
This is a useful way to include in the theme the CSS scripts that are hosted in the content delivery network like bootstrap, jquery, font awesome, and so on.
But during my research, I found bloginfo() function is able to load internal stylesheet with a single code line.
Let’s see how to use bloginfo too add stylesheet.
Add a stylesheet by bloginfo() function
To include the stylesheet using bloginfo, write a stylesheet link tag in the header.php file or index.php and not the functions.php file.
Here are the few steps to add style.css using bloginfo function.
Open header.php or index.php
Move to the head tag (<head>) and write the stylesheet link tag.
<head>
<link rel="stylesheet" href="style.css">
</head>
Set href attribute to <?php bloginfo(‘stylesheet_url’);?>, thus the link element changes to <link rel="stylesheet" href="<?php bloginfo('stylesheet_url');?>">
If you go back to the page frontend you will see again your page design layout as it was defined in the local CSS file.
Why you should use wp_enqueue_style
In this post, there are three ways you can use to include the stylesheet.
You can add the stylesheet by writing the link tag directly without using any WordPress function.
But why it is recommended to use wp_enqueue_style instead?
The short answer is to avoid stylesheet duplication. wp_enqueue_style checks if the provided stylesheet exists or not. If the CSS URI exists, the function won’t over-write the same stylesheet.
Duplicating the CSS scripts may cause a slow page loading. This impacts a blog page in the search engine rankings negatively.
Stylesheet over-writing happens when you use CSS frameworks that are hosted on CDN servers.
If a theme user installs a plugin, and the plugin uses the same stylesheet Uri, the plugin will duplicate CSS scripts.
For example,
The bootstrap CDN is https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css, which is used largely by website designers and developers.
Then, I include bootstrap CDN of bootstrap.min.css without wp_enqueue_style.
<link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
Some WordPress plugin developers include bootstrap CDN for the plugin.
So, when I download that plugin, it is going to include another <link rel="stylesheet" href="https://stackpath.bootstrapcdn.com/bootstrap/4.5.2/css/bootstrap.min.css">
in the head tag.
But using wp_enqueue_style
prevents this duplication.
Why your wp_enqueue_style is not working
Sometimes you may find wp_enqueue_style doesn’t work. So, it fails to add the stylesheet.
Basing on StackOverflow questions, here are the main reasons why wp_enqueue_style fails to load the stylesheet.
wp_head is not included in the header.php
If the stylesheet is not added to the head tag, there is a probability wp_head has not been included in the header.php file.
Therefore, you should include wp_head by writing this line <?php wp_head();?>
to solve the issue.
Writing wp_enqueue_style in functions.php only is not enough to load the script.
Incorrect stylesheet path
If the stylesheet path is not passed correctly or the CSS file Uri is not provided at all, the wp_enqueue_style function won’t render a stylesheet.
To fix this, check very carefully a file Uri source and also, use the correct WordPress functions to retrieve the file path.
For example, if the stylesheet mycustome.css file is in the my-styles-folder directory. The correct function is get_template_directory_uri.
The correct function to retrieve the file path is get_template_directory_uri().’my-styles-folder/mycustome.css’
Therefore, calling wp_enqueue_style('mystyle',get_template_directory_uri().'/my-styles-folder/mycustome.css')
will add the CSS stylesheet without any error.
Wrap-up
bloginfo(‘stylesheet_url’) and wp_enqueue_style are the two WordPress functions that can be used to add a stylesheet. wp_enqueue_style can render internal and external stylesheet URL. It cannot duplicate stylesheet when a plugin tries to add the same stylesheet. wp_enqueue_style receives five arguments but the two arguments which specify stylesheet name and style path are the keys to load CSS script. And wp_head should be called in the header.php.
bloginfo(‘stylesheet_url’) can be written in link tag’s href attribute without using wp_head but it cannot stop stylesheet over-writing.